GroveStreams Java - Quick Start
This tutorial will walk through the creation of a GroveStreams user account, an organization, and Java code that uploads random numbers.
Step 1: Create a Free GroveStreams User Account and Organization
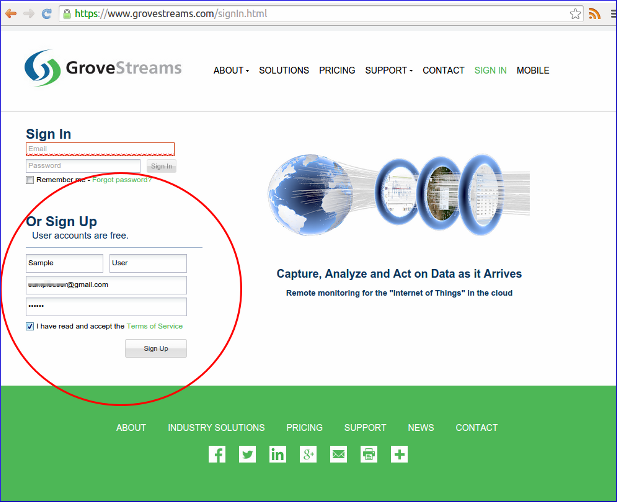
Open a browser and navigate to the GroveStreams registration page and sign up.
Log into GroveStreams after creating your free user account.
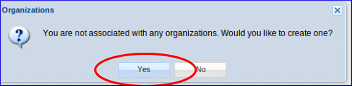
You will be prompted to create an organization. Select Yes.
An organization is a Workspace, typically representing a home, business, or organization. Each organization has its own set of components, streams, dashboards, maps, and other items. An organization allows you to control user and device access to the organization. You are automatically the "owner" and given full access rights when you create an organization. Other users may invite you to their organizations with rights they give you. All of the organizations, you "own" or are a member of, will appear in your GroveStreams start page (the first page that appears when you sign in).
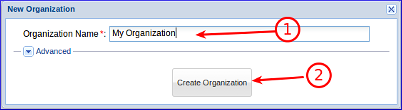
1. Enter a name for the new organization. It can be any name.
2. Click Create Organization.
3. Enter your new organization by clicking on its name.
Step 2: Find your GroveStreams Secret API key for your organization
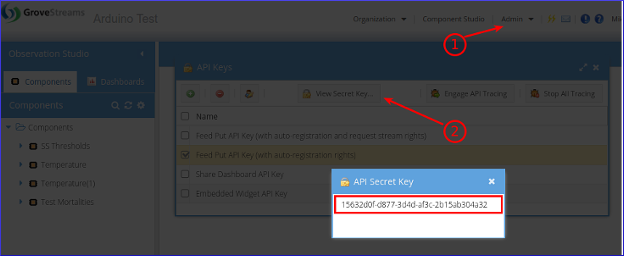
To find your Secret API Key:
1. Select the Admin - API Keys menu option and choose the Feed Put API Key (with auto-registration rights) key
2. Click View Secret Key
3. Select and copy the API Secret Key to the clipboard (Ctrl-c) when you are ready to paste it below.
Step 3: Create the Java Stream Feeder
The GroveStreams API is a Restful API and can be used by most programming languages. For this example we will use the Java programming language and we will use the simple feed PUT API which does not involve any JSON.
We highly recommend downloading and installing a free Java editor such as Eclipse.
1. Download and run Eclipse
2. Create a Java Project in Eclipse
3. Create a Java class called HelloWorld and copy the code below to this new class file
4. Right click on your Java Project and choose Build Path -
Configure Build Path, click on the libraries tab, choose Add
JARs (or Add External JARs, depending on where you placed
the required libraries) and add the required library jars
Required Libraries
commons-codec-1.4.jar
commons-httpclient-3.1.jar
commons-logging-1.1.1.jar
Code (Don't forget to replace the api_key token in
the code below with your Secret API Key!)
import java.io.IOException;
import java.util.Random;
import org.apache.commons.httpclient.HttpClient;
import org.apache.commons.httpclient.HttpStatus;
import
org.apache.commons.httpclient.methods.PutMethod;
public class HelloWorld {
private static final
String api_key = "YOUR_SECRET_API_KEY_HERE";
//Change This!!!
private static final String
component_id = "myOrg.helloWorld";
public static
void main(String[] args) {
PutMethod putMethod =
null;
try {
//
Connect to GroveStreams
HttpClient httpClient =
new HttpClient();
while (true) {
Random rand = new Random();
String url =
String.format("http://grovestreams.com/api/feed?api_key=%s&compId=%s&random=%f",
api_key, component_id, rand.nextFloat());
putMethod = new PutMethod(url);
putMethod.setRequestHeader("Accept", "application/json");
putMethod.setRequestHeader("Content-type",
"application/json");
//Upload the
sample
int statusCode =
httpClient.executeMethod(putMethod);
if
(statusCode != HttpStatus.SC_OK) {
System.out.println("Upload to grovestreams.com failure: " +
putMethod.getStatusLine().toString());
}
else {
System.out.println("Upload to
grovestreams.com succeeded");
}
//Sleep 10 seconds
Thread.sleep(10000);
}
}
catch (IOException e) {
System.out.println(e.getMessage());
} catch
(InterruptedException e) {
System.out.println(e.getMessage());
} finally {
// Release the connection
if
(putMethod != null) {
putMethod.releaseConnection();
}
}
}
}
Step 6: Run your stream feeder
The easiest way to run your feeder is to run it from within Eclipse.
Right click your HelloWorld class and and choose Debug As - Java Application
Step 7: See Your Results
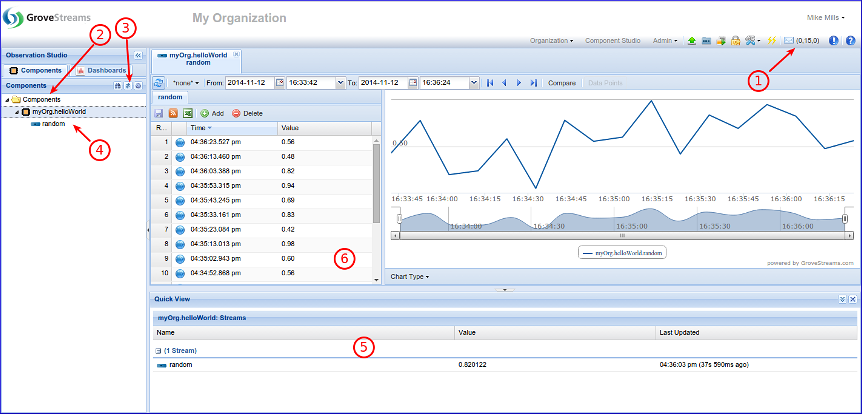
1. The notification button will indicate if you have any notifications. Some notifications report errors. Check for any notifications that may contain any upload errors.
2. Select the Components folder.
3. Click the Refresh button.
4. Your new component will appear under the Components folder. Select and double-click a stream.
5. The stream's latest measurements will appear within the Quick View panel. The Quick View panel will display No Data if no measurements have been uploaded..
6. Stream Viewer. Explore a stream's historical measurments and it's latest measurements. Select different cycles to zoom in or out.
Congratulations! You have created a simple Java feeder!
Click on the Dashboards tab to create dashboards and maps. Right click on the component and choose Edit Component to set your component's location, add stream units, create events with notifications, and change other properties. Keep exploring and learning!
Troubleshooting
Watch for errors within the Eclipse console pane. Successful API calls to GroveStreams should return a status of: HTTP/1.1 200 OK. The console will show "Upload to grovestreams.com succeeded".If the call made it to a GroveStreams server, but an error still occurred, a GroveStreams system notification will be created that contains the error. While in the GroveStreams organization's Observation Studio, click on the Notifications button located in the top toolbar (It looks like an envelope) and select the System tab. Click Refresh to list new notifications.
Any parameter that is part of the URL within the Agent needs to be URL encoded. This should only become an issue if you have given any parameters a value with reserved URL characters (such as spaces or commas). There are many free URL encoders on-line that you can use to encode your URL or URL parameters such as freeformatter.com.
Ensure you are not using the same API Secret Key for different organizations. API Secret Keys are only associated with the organization they have been created within and can only be used for that organization.
Troubleshooting Checklist
- Did you remember to change the api_key value within the Java code above?
- Have you checked your GroveStreams organization for any notifications?
- Is the Eclipse console showing errors?
- Still can't figure out what's wrong? Delete your GroveStreams organization, start over, and follow the instructions carefully. Sometimes it's easier and faster to start over than to try and figure out what step was missed or performed incorrectly.
Still having problems or you wish to modify the solution and are not sure what to do?
Post a question on our forum or send us an email and we'll do our best to help you out: support@grovestreams.comHelpful Links
GroveStreams Help CenterGroveStreams Simple Feed PUT API
GroveStreams Forum